UVM Register Abstraction Layer (RAL) - overview
Within an UVM testbench a register model is used
either as a means of looking up a mirror of the current DUT hardware state
or as means of accessing the hardware via the front or back door
and updating the register model database.
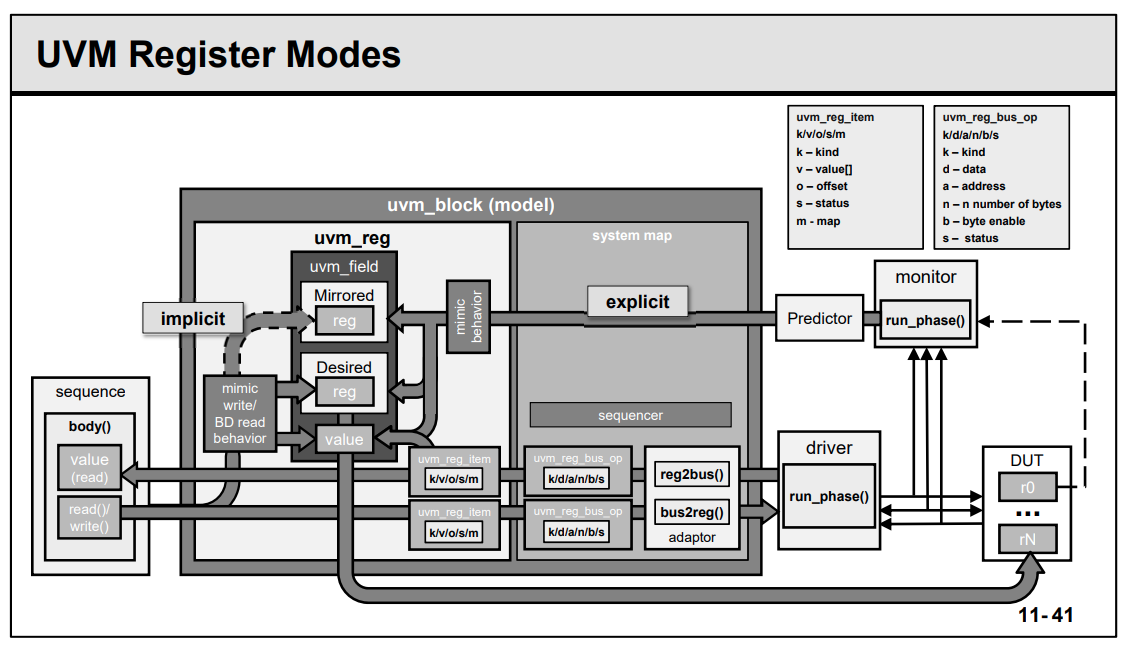
Register Frontdoor Write
1 | model.r0.write(status, value, [UVM_FRONTDOOR], .parent(this)); |
- Sequence sets
uvm_reg
with value uvm_reg
content is translated into bus transaction- Driver gets bus transaction and writes DUT register
- Mirror can be updated either implicitly or explicitly (predictor)
Register Backdoor Write
1 | model.r0.write(status, value, UVM_BACKDOOR, .parent(this)); |
uvm_reg
uses DPI/XMR to set DUT register with value
- Physical interface is bypassed
- Register behavior is mimicked
Register Backdoor Poke
1 | model.r0.poke(status, value, .parent(this)); |
uvm_reg
used DPI/XMR to set DUT register with
value as is
- Physical interface is bypassed
- Register behavior is NOT mimicked
Register Frontdoor Read
1 | model.r0.read(status, value, [UVM_FRONTDOOR], .parent(this)); |
- Sequence executes
uvm_reg
READ uvm_reg
is translated into bus transaction- Driver gets bus transaction and read DUT register
- Read value is translated into
uvm_reg
data and returned to sequence - Mirror updates
Register Backdoor Read
1 | model.r0.read(status, value, UVM_BACKDOOR, .parent(this)); |
uvm_reg
used DPI/XMR to get DUT register value
- Physical interface is bypassed
- Register behavior is mimicked (acc)
1 | // EXECUTE READ... |
Register Backdoor Peek
1 | model.r0.peek(status, value, .parent(this)); |
uvm_reg
uses DPI/XMR to get DUT register value
as is
- Physical interface is bypassed
- Register behavior is NOT mimicked
1 | task uvm_reg::peek(output uvm_status_e status, |
Methods to handle property of uvm_reg or uvm_reg_block
mirror
1 | model.mirror(status, [check], [path], .parent(this)); |
Update mirrored and desired properties with DUT content
set
1 | model.r0.set(value); |
Set value in desired properties
randomize
1 | model.randomize(); |
Populate desired property with random value
get
1 | value = model.r0.get(); |
Get value from desired property
update
1 | model.update(status, [path], .parent(this)); |
Update DUT and mirrored property with desired property if mirrored property is different from desired
predict
1 | model.r0.predict(value); |
Set the value of mirrored property
get_mirrored_value
1 | value = model.r0.get_mirrored_value(); |
Get value from mirrored property
Backdoor Access
- Two ways to generate the backdoor access:
- Via SystemVerilog Cross Module Reference (XMR)
- Via SystemVerilog DPI call
- Both allow register model to be part of SystemVerilog package
- XMR implementation is faster
- Requires user to compile one additional file and at compile-time provide top level path to DUT
- VCS only
- DPI implementation is slower
- No additional file is needed and top level path can be provided at run-time
- Portable to other simulators
UVM Register Classes: uvm_reg_bus_op & uvm_reg_item
The generic register item is implemented as a struct in order to
minimise the amount of memory resource it uses. The struct is defined as
type uvm_reg_bus_op
and this contains 6 fields:
Property | Type | Comment/Description |
---|---|---|
addr | uvm_reg_addr_t | Address field, defaults to 64 bits |
data | uvm_reg_data_t | Read or write data, defaults to 64 bits |
kind | uvm_access_e | UVM_READ or UVM_WRITE |
n_bits | unsigned int | Number of bits being transferred |
byte_en | uvm_reg_byte_en_t | Byte enable |
status | uvm_status_e | UVM_IS_OK, UVM_IS_X, UVM_NOT_OK |
1 | typedef struct { |
1 | class uvm_reg_item extends uvm_sequence_item; |
Registers
The register class contains a build
method which is used
to create and configure the
fields.
this
build
method is not called by the UVMbuild_phase
, since the register is anuvm_object
rather than anuvm_component
1 | // |
1 | // Function: new |
As shown above, Register width is 32 same with the bus width, lower 14 bit is configured.
RTL
1 |
|
Register Maps
Two purpose of the register map
- provide information on the offset of the registers, memories and/or register blocks
- identify bus agent based sequences to be executed ???
There can be several register maps within a block, each one can specify a different address map and a different target bus agent
register map has to be created which the register block using the
create_map
method
1 | // |
The n_bytes parameter is the word size (bus width) of the bus to which the map is associated. If a register’s width exceeds the bus width, more than one bus access is needed to read and write that register over that bus.
he byte_addressing argument affects how the address is incremented in these consecutive accesses. For example, if n_bytes=4 and byte_addressing=0, then an access to a register that is 64-bits wide and at offset 0 will result in two bus accesses at addresses 0 and 1. With byte_addressing=1, that same access will result in two bus accesses at addresses 0 and 4.
The default for byte_addressing is 1
The first map to be created within a register block is assigned to the default_map member of the register block
Register Adapter
uvm_reg_adapter | |
---|---|
Methods | Description |
reg2bus | Overload to convert generic register access items to target bus agent sequence items |
bus2reg | Overload to convert target bus sequence items to register model items |
Properties (Of type bit) | Description |
supports_byte_enable | Set to 1 if the target bus and the target bus agent supports byte enables, else set to 0 |
provides_responses | Set to 1 if the target agent driver sends separate response sequence_items that require response handling |
The provides_responses bit should be set if the agent driver returns a separate response item (i.e.
put(response)
, oritem_done(response)
) from its request item
Prediction
the update, or prediction, of the register model content can occur using one of three models
Auto Prediction
This mode of operation is the simplest to implement, but suffers from the drawback that it can only keep the register model up to date with the transfers that it initiates. If any other sequences directly access the target sequencer to update register content, or if there are register accesses from other DUT interfaces, then the register model will not be updated.
1 | function void uvm_reg_map::set_auto_predict(bit on=1); m_auto_predict = on; endfunction |
// Function: set_auto_predict
//
// Sets the auto-predict mode for his map.
//
// When on is TRUE,
// the register model will automatically update its mirror (what it thinks should be in the DUT)
immediately after any bus read or write operation via this map. Before a
uvm_reg::write
// or
uvm_reg::read
operation returns, the register’suvm_reg::predict
method is called to updatethe mirrored value in the register.
//
// When on is FALSE, bus reads and writes via this map do not
// automatically update the mirror. For real-time updates to the mirror
// in this mode, you connect a
uvm_reg_predictor
instance to the bus// monitor. The predictor takes observed bus transactions from the
// bus monitor, looks up the associated
uvm_reg
register given// the address, then calls that register’s
uvm_reg::predict
method.// While more complex, this mode will capture all register read/write
// activity, including that not directly descendant from calls to
//
uvm_reg::write
anduvm_reg::read
.//
// By default, auto-prediction is turned off.
//

The register model content is updated based on the register accesses it initiates
Explicit Prediction (Recommended Approach)
Explicit prediction is the default mode of prediction

The register model content is updated via the predictor
component based on all observed bus transactions, ensuring that
register accesses made without the register model are mirrored
correctly. The predictor looks up the accessed register by address then
calls its predict()
method
uvm_reg::predict & uvm_reg::do_predict
1 | // predict |
uvm_reg_field::predict & uvm_reg_field::do_predict
1 | // predict |
uvm_access_e
1 | // Enum: uvm_access_e |
uvm_predict_e
1 | // Enum: uvm_predict_e |
uvm_path_e
1 | // Enum: uvm_path_e |